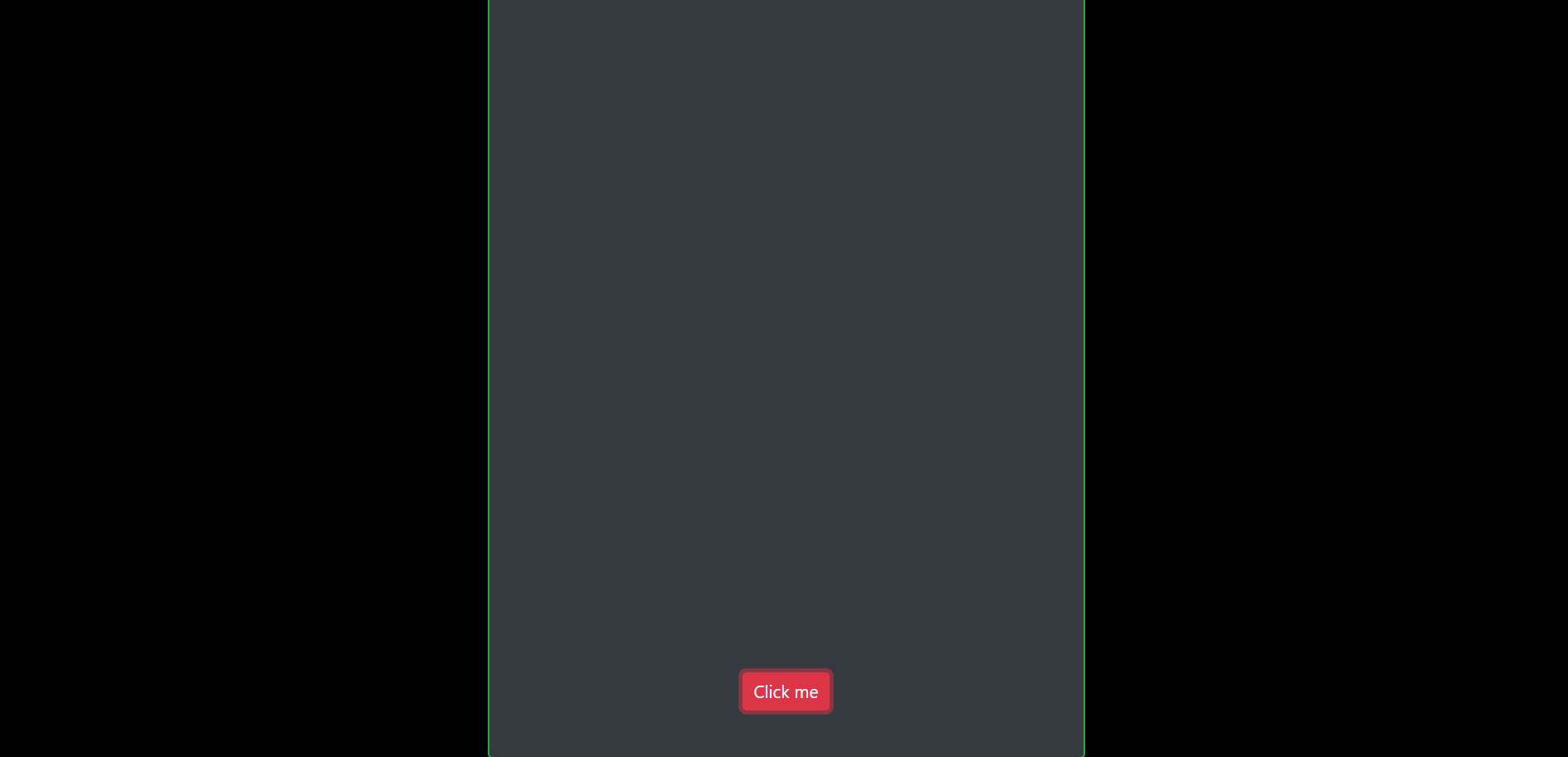
How to scroll to top on button click?
When we are at the bottom of our page instead of scrolling mouse it is very easy and user-friendly to have a button to scroll to top with a single click. We can accomplish scroll to top on button click with jQuery very easily.
HTML :
<!DOCTYPE html>
<html>
<head>
<title>Scroll to top</title>
<link href="//maxcdn.bootstrapcdn.com/bootstrap/4.1.1/css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css">
<script src="//maxcdn.bootstrapcdn.com/bootstrap/4.1.1/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.5.0/css/all.css">
</head>
<body>
<div class="container" id="main">
<div class="card card-login mx-auto text-center bg-dark">
<div class="card-header mx-auto bg-dark">
<span> </span><br/>
<span class="logo_title mt-5"> Scroll </span>
</div>
<div class="card-body">
<div id="div1" class="scrollDiv">
Scrolling...
</div>
<br/>
<div id="div2" class="scrollDiv">
Scrolling...
</div>
<button id="click" class="btn btn-sm btn-danger">Click me</button>
</div>
</div>
</div>
</body>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.5.1/jquery.min.js"></script>
</html>
- Attach a button at the bottom of the page.
- Call the jQuery script to scroll to top on button click.
Here I included some Bootstrap, jQuery and fontawesome links as follows,
Bootstrap css: https://maxcdn.bootstrapcdn.com/bootstrap/4.1.1/css/bootstrap.min.css
Bootstrap js : https://maxcdn.bootstrapcdn.com/bootstrap/4.1.1/js/bootstrap.min.js
Fontawesome : https://use.fontawesome.com/releases/v5.5.0/css/all.css
jQuery js : https://ajax.googleapis.com/ajax/libs/jquery/1.5.1/jquery.min.js
Include below internal CSS to obtain the demo design
<style type="text/css">
body {
background: #000 !important;
}
.card {
border: 1px solid #28a745;
}
.card-login {
margin-top: 50px;
padding: 18px;
max-width: 30rem;
}
.card-header {
color: #fff;
/*background: #ff0000;*/
font-family: sans-serif;
font-size: 20px;
font-weight: 600 !important;
margin-top: 10px;
border-bottom: 0;
}
.input-group-prepend span{
width: 50px;
background-color: #ff0000;
color: #fff;
border:0 !important;
}
input:focus{
outline: 0 0 0 0 !important;
box-shadow: 0 0 0 0 !important;
}
.login_btn{
width: 130px;
}
.login_btn:hover{
color: #fff;
background-color: #ff0000;
}
.btn-outline-danger {
color: #fff;
font-size: 18px;
background-color: #28a745;
background-image: none;
border-color: #28a745;
}
.form-control {
display: block;
width: 100%;
height: calc(2.25rem + 2px);
padding: 0.375rem 0.75rem;
font-size: 1.2rem;
line-height: 1.6;
color: #28a745;
background-color: transparent;
background-clip: padding-box;
border: 1px solid #28a745;
border-radius: 0;
transition: border-color 0.15s ease-in-out, box-shadow 0.15s ease-in-out;
}
.input-group-text {
display: -ms-flexbox;
display: flex;
-ms-flex-align: center;
align-items: center;
padding: 0.375rem 0.75rem;
margin-bottom: 0;
font-size: 1.5rem;
font-weight: 700;
line-height: 1.6;
color: #495057;
text-align: center;
white-space: nowrap;
background-color: #e9ecef;
border: 1px solid #ced4da;
border-radius: 0;
}
.scrollDiv
{
height: 1000px;
width: 100px;
color:#ffffff;
}
</style>
jQuery :
<script>
$(document).ready(function (){
$("#click").click(function (){
$('html, body').animate({
scrollTop: $("#main").offset().top
}, 2000);
});
});
</script>
- When the button with id click clicked an animation will happen which will scroll the position to top of the page.
- animate() will perform a custom animation of a set of CSS properties.
- scrollTop() will get the current vertical position of the scroll bar for the first element in the set of matched elements or set the vertical position of the scroll bar for every matched element.
- offset() will get the current coordinates of the first element, or set the coordinates of every element, in the set of matched elements, relative to the document.
Here we go with the solution to scroll to top on button click.
Hope this tutorial for scroll to top on button click will help you.
For more jQuery soltions please visit jQuery