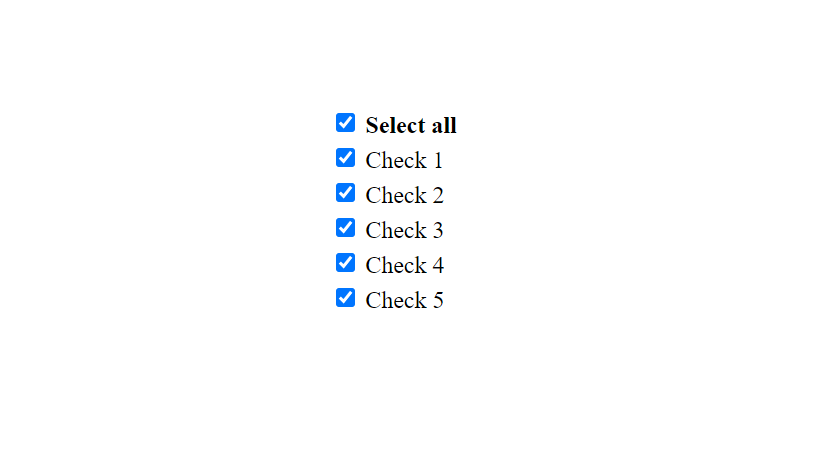
How to do select all checkbox using jQuery. Here we’ll discuss both select all and deselect all checkbox using jQuery.
Most of the time we may need to implement select all or deselect all checkbox. To do this the easiest and simple way is using jQuery. This tutorial will help you to complete the task.
OUTPUT :-
tr { border:none!important; }
Select all |
---|
Check 1 |
Check 2 |
Check 3 |
Check 4 |
Check 5 |
$(document).ready(function(){
$(‘#select_all’).on(‘click’,function(){
if(this.checked){
$(‘.check’).each(function(){
this.checked = true;
});
}else{
$(‘.check’).each(function(){
this.checked = false;
});
}
});
$(‘.check’).on(‘click’,function(){
if($(‘.check:checked’).length == $(‘.check’).length){
$(‘#select_all’).prop(‘checked’,true);
}else{
$(‘#select_all’).prop(‘checked’,false);
}
});
});
HTML :-
<table>
<tr>
<th><input type="checkbox" id="select_all"/> Select all</th>
</tr>
<tr>
<td><input type="checkbox" class="check" value="1"/> Check 1</td>
<td><input type="checkbox" class="check" value="2"/> Check 2</td>
<td><input type="checkbox" class="check" value="3"/> Check 3</td>
<td><input type="checkbox" class="check" value="4"/> Check 4</td>
<td><input type="checkbox" class="check" value="5"/> Check 5</td>
</tr>
</table>
jQuery :-
<script type="text/javascript">
$(document).ready(function(){
$('#select_all').on('click',function(){
if(this.checked){
$('.check').each(function(){
this.checked = true;
});
}else{
$('.check').each(function(){
this.checked = false;
});
}
});
$('.check').on('click',function(){
if($('.check:checked').length == $('.check').length){
$('#select_all').prop('checked',true);
}else{
$('#select_all').prop('checked',false);
}
});
});
</script>
jQuery : https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js
For other jQuery solutions please visit jQuery